CellML API
cellml_services::CodeGenerator Interface Reference
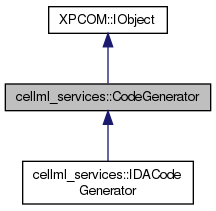
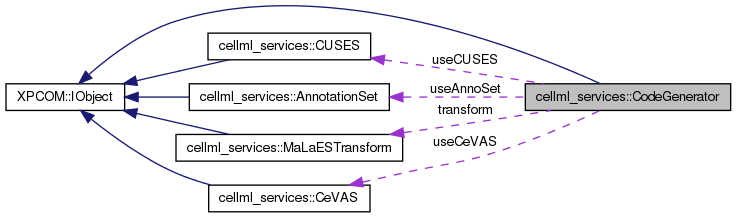
Public Member Functions | |
CodeInformation | generateCode (in cellml_api::Model sourceModel) |
Generates a CodeInformation object for a model. | |
CustomGenerator | createCustomGenerator (in cellml_api::Model sourceModel) |
Creates a CustomGenerator object for a model. | |
![]() | |
void | add_ref () |
Called to indicate that some code is keeping an owning reference to the object. | |
oneway void | release_ref () |
Removes a reference to an object which was created by some other means (e.g. | |
IObject | query_interface (in utf8string id) |
Returns an IObject of the same implementation, which supports a specific interface. |
Public Attributes | |
attribute wstring | constantPattern |
The pattern used to generate constant names (if they are not already annotated). | |
attribute wstring | stateVariableNamePattern |
The pattern used to generate state variable names (if they are not already annotated). | |
attribute wstring | algebraicVariableNamePattern |
The pattern used to generate algebraic variable names (if they are not already annotated). | |
attribute wstring | rateNamePattern |
The pattern used to generate rate names (if they are not already annotated). | |
attribute wstring | voiPattern |
The pattern used to generate variable of integration names (if they are not already annotated). | |
attribute wstring | sampleDensityFunctionPattern |
The pattern used to generate code for sampling from a density function. | |
attribute wstring | sampleRealisationsPattern |
The pattern used to generate code for sampling using realisations. | |
attribute wstring | boundVariableName |
The name to use for the locally bound variable given to the probability density function in the generated code. | |
attribute unsigned long | arrayOffset |
The offset at which array indices start. | |
attribute wstring | assignPattern |
The pattern which is used to set variables by assignment. | |
attribute wstring | solvePattern |
The pattern which is used to compute variables which cannot be set by assignment. | |
attribute wstring | solveNLSystemPattern |
The pattern which is used to solve a system of n equations in n unknowns. | |
attribute wstring | temporaryVariablePattern |
The pattern which is used to determine the name of temporary variables when they are required for infinitesimally expressions. | |
attribute wstring | declareTemporaryPattern |
The pattern used to declare that a temporary variable is in use. | |
attribute wstring | conditionalAssignmentPattern |
The pattern used when a top-level piecewise statement is encountered. | |
attribute cellml_services::MaLaESTransform | transform |
A MaLaES transform to use. | |
attribute cellml_services::CeVAS | useCeVAS |
The CeVAS association to use. | |
attribute cellml_services::CUSES | useCUSES |
The CUSES object to use. | |
attribute cellml_services::AnnotationSet | useAnnoSet |
The annotation set to use. | |
attribute boolean | allowPassthrough |
If false, any text entered shall be passed through the MaLaES sanitiser to strip out passthrough text. | |
![]() | |
readonly attribute string | objid |
Fetches the ID of the object. |
Detailed Description
Member Function Documentation
CustomGenerator cellml_services::CodeGenerator::createCustomGenerator | ( | in cellml_api::Model | sourceModel | ) |
Creates a CustomGenerator object for a model.
Note that this picks up on the transform, CeVAS, CUSES, AnnoSet, stateVariableNamePattern, assignPattern, solvePattern, solveNLSystemPattern, and arrayOffset - everything else is specific to generateCode.
CodeInformation cellml_services::CodeGenerator::generateCode | ( | in cellml_api::Model | sourceModel | ) |
Generates a CodeInformation object for a model.
- Parameters
-
sourceModel The model for which to generate the CodeInformation.
Member Data Documentation
attribute wstring cellml_services::CodeGenerator::algebraicVariableNamePattern |
attribute boolean cellml_services::CodeGenerator::allowPassthrough |
If false, any text entered shall be passed through the MaLaES sanitiser to strip out passthrough text.
This prevents the generated code from containing untrusted code from an untrusted model (depending on the target language and the MaLaES definitions used).
If true, no such sanitisation shall be performed, and it is possible to inject arbitrary code from the model into the generated code by using a MathML csymbol element like the following: <mathml:csymbol definitionURL="http://www.cellml.org/tools/api#passthrough"> code-to-inject</mathml:csymbol>
Default: false
attribute unsigned long cellml_services::CodeGenerator::arrayOffset |
attribute wstring cellml_services::CodeGenerator::assignPattern |
attribute wstring cellml_services::CodeGenerator::boundVariableName |
attribute wstring cellml_services::CodeGenerator::conditionalAssignmentPattern |
The pattern used when a top-level piecewise statement is encountered.
The generated output treats the first case specially, and this case has the condition and statement outside of <CASES>. The generated output for the remaining cases is produced by repeating the pattern between <CASES> and </CASES>. Default: if (<CONDITION>)
{
<STATEMENT>
}
<CASES>else if (<CONDITION>)
{
<STATEMENT>
}
</CASES>
attribute wstring cellml_services::CodeGenerator::constantPattern |
attribute wstring cellml_services::CodeGenerator::declareTemporaryPattern |
attribute wstring cellml_services::CodeGenerator::rateNamePattern |
attribute wstring cellml_services::CodeGenerator::sampleDensityFunctionPattern |
The pattern used to generate code for sampling from a density function.
can be used to split the code into a main and supplementary part. <EXPR> will be substituted for the expression for the probability density function. <ID> will be substituted for a number which is unique for each instance in which this pattern is used (within that substitution). Default: SampleUsingPDF(&pdf_<ID>, CONSTANTS, ALGEBRAIC)double pdf_<ID>(double bvar, double* CONSTANTS, double* ALGEBRAIC)
{
return (<EXPR>);
}
attribute wstring cellml_services::CodeGenerator::sampleRealisationsPattern |
The pattern used to generate code for sampling using realisations.
<numChoices> will be substituted for the number of realisations to choose between. The code between <eachChoice>...</eachChoice> will be substituted for each of the choices. In this region, <choiceNumber> gives the zero-based index of the choice, <choiceAssignments> gives the assignments that are required when the choice is selected. Default: switch (rand() % <numChoices>)
{
<eachChoice>case <choiceNumber>:
<choiceAssignments>break;
</eachChoice>}
attribute wstring cellml_services::CodeGenerator::solveNLSystemPattern |
The pattern which is used to solve a system of n equations in n unknowns.
<EXPR> is replaced by the expression for the difference between the two sides of the equality. causes the following text to go into the supplementary function array instead of the main code list. gives the name to the variable to compute. <ID> is replaced with a numeric ID unique to this equation.
attribute wstring cellml_services::CodeGenerator::solvePattern |
The pattern which is used to compute variables which cannot be set by assignment.
<LHS> is replaced by the expression for the first argument to the equality. <RHS> is replaced by the expression for the second argument to the equality. causes the following text to go into the supplementary function array instead of the main code list. gives the name to the variable to compute. <ID> is replaced with a numeric ID unique to this equation. Default: rootfind_<ID>(VOI, CONSTANTS, RATES, STATES, ALGEBRAIC, pret);
void objfunc_<ID>(double <em>p, double *hx, int m, int n, void *adata)
{
struct rootfind_info rfi = (struct rootfind_info*)adata;
#define VOI rfi->aVOI
#define CONSTANTS rfi->aCONSTANTS
#define RATES rfi->aRATES
#define STATES rfi->aSTATES
#define ALGEBRAIC rfi->aALGEBRAIC
#define pret rfi->aPRET
= p;
*hx = (<LHS>) - (<RHS>);
#undef VOI
#undef CONSTANTS
#undef RATES
#undef STATES
#undef ALGEBRAIC
#undef pret
} rootfind_<ID>(double VOI, double CONSTANTS, double* RATES, double* STATES, double* ALGEBRAIC, int* pret)
{
static double val = <IV>;
double bp, work[LM_DIF_WORKSZ(1, 1)];
struct rootfind_info rfi;
rfi.aVOI = VOI;
rfi.aCONSTANTS = CONSTANTS;
rfi.aRATES = RATES;
rfi.aSTATES = STATES;
rfi.aALGEBRAIC = ALGEBRAIC;
rfi.aPRET = pret;
do_levmar(objfunc_<ID>, &val, &bp, work, pret, 1, &rfi);
= val;
}
attribute wstring cellml_services::CodeGenerator::stateVariableNamePattern |
attribute wstring cellml_services::CodeGenerator::temporaryVariablePattern |
attribute cellml_services::MaLaESTransform cellml_services::CodeGenerator::transform |
A MaLaES transform to use.
If will be null if it has not been set, and no code has been generated from this generator. If generateCode is called but this is null, a new default MaLaESTransform will be created, with a MAL description suitable for use in C programs. Default: null
attribute cellml_services::AnnotationSet cellml_services::CodeGenerator::useAnnoSet |
attribute cellml_services::CeVAS cellml_services::CodeGenerator::useCeVAS |
The CeVAS association to use.
If this is null (the default) a new CeVAS will be requested every time you call generateCode. Be careful to use a CeVAS which corresponds to the exact model being passed in to generateCode. The model cannot be changed once the CeVAS is created, until the CeVAS is destroyed. Default: null
attribute cellml_services::CUSES cellml_services::CodeGenerator::useCUSES |
The CUSES object to use.
If this is null (the default) a new CUSES will be requested every time you call generateCode. Be careful to use a CUSES which corresponds to the exact model being passed in to generateCode. The model cannot be changed once the CUSES is created, until the CUSES is destroyed. Default: null