CellML API
cellml_api::CellMLElement Interface Reference
This is a general interface from which all CellML elements inherit. More...
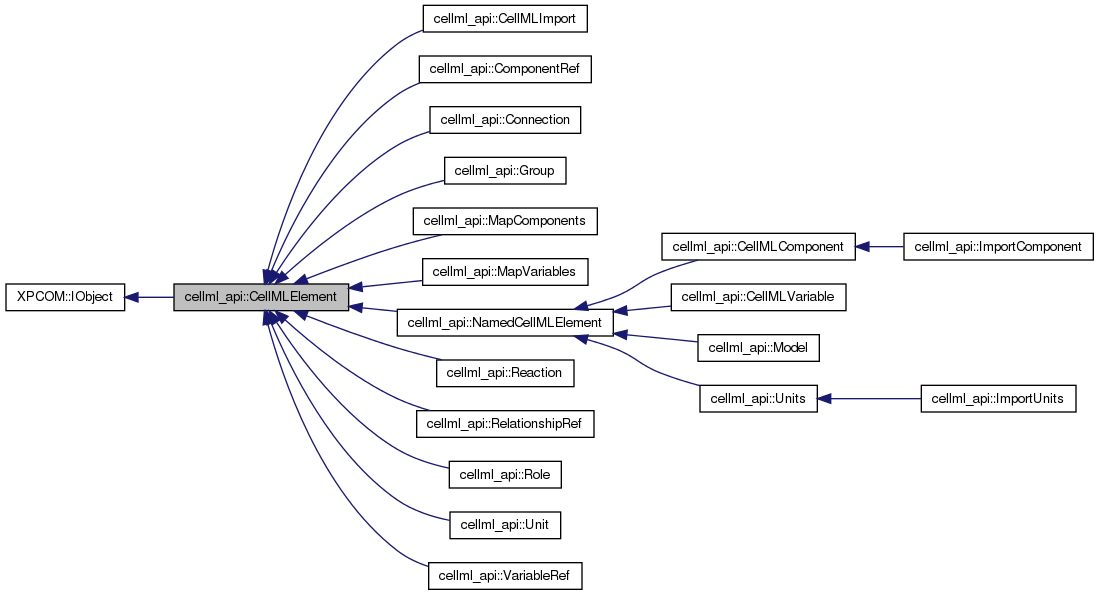
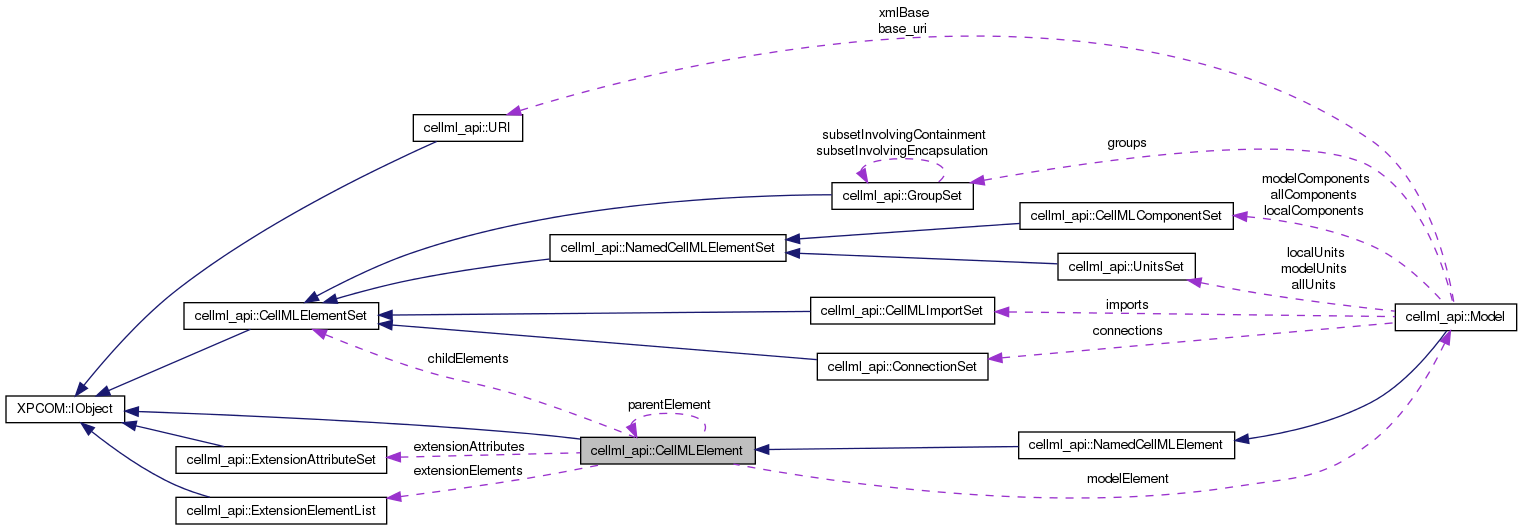
Public Member Functions | |
void | insertExtensionElementAfter (in ExtensionElement marker, in ExtensionElement newEl) |
Insert an element into the collection of extension elements without disturbing the order of the existing elements. | |
void | appendExtensionElement (in ExtensionElement x) |
Equivalent to insertExtensionElementAfter(last extension element, x). | |
void | prependExtensionElement (in ExtensionElement x) |
Equivalent to insertExtensionElementAfter(nil, x) | |
void | removeExtensionElement (in ExtensionElement x) |
Remove an extension element. | |
void | replaceExtensionElement (in ExtensionElement x, in ExtensionElement y) |
Find an extension element, and if it is found, replace it with another element. | |
void | clearExtensionElements () |
Remove all extension elements from this element. | |
void | addElement (in CellMLElement x) raises (CellMLException) |
Add a CellML element to this element. | |
void | removeElement (in CellMLElement x) |
Removes a CellML element from this element. | |
void | replaceElement (in CellMLElement x, in CellMLElement y) raises (CellMLException) |
Removes a CellML element from this element, and replaces it with another CellML element. | |
void | removeByName (in CellMLAttributeString type, in CellMLAttributeString name) |
Remove a CellML element by name, or do nothing if that element is not found. | |
void | setUserData (in wstring key, in UserData data) |
Sets user-supplied annotations on this element. | |
UserData | getUserData (in wstring key) raises (CellMLException) |
Retrieves user-supplied annotations previously set on this element. | |
CellMLElement | clone (in boolean deep) |
Clones a CellMLElement, and optionally all children. | |
wstring | getExtensionAttributeNS (in wstring ns, in wstring localName) |
Fetches an extension attribute. | |
void | setExtensionAttributeNS (in wstring ns, in wstring qualifiedName, in wstring value) |
Sets an extension attribute (adding it if it doesn't already exist, otherwise replacing it). | |
void | removeExtensionAttributeNS (in wstring ns, in wstring localName) |
Removes an extension attribute. | |
![]() | |
void | add_ref () |
Called to indicate that some code is keeping an owning reference to the object. | |
oneway void | release_ref () |
Removes a reference to an object which was created by some other means (e.g. | |
IObject | query_interface (in utf8string id) |
Returns an IObject of the same implementation, which supports a specific interface. |
Public Attributes | |
readonly attribute CellMLAttributeString | cellmlVersion |
The CellML version that this element corresponds to. | |
attribute CellMLAttributeString | cmetaId |
This element's cmeta id (which may be defined on any CellML element). | |
readonly attribute ExtensionElementList | extensionElements |
The collection of extension elements associated with this CellML element. | |
readonly attribute CellMLElementSet | childElements |
Get a list of all the child CellMLElements in this element. | |
readonly attribute CellMLElement | parentElement |
The parent element of this element... | |
readonly attribute Model | modelElement |
The underlying model element. | |
readonly attribute ExtensionAttributeSet | extensionAttributes |
Fetches the set of all extension attributes, which can be used to iterate through the extension attributes. | |
![]() | |
readonly attribute string | objid |
Fetches the ID of the object. |
Detailed Description
This is a general interface from which all CellML elements inherit.
Definition at line 164 of file CellML_APISPEC.idl.
Member Function Documentation
void cellml_api::CellMLElement::addElement | ( | in CellMLElement | x | ) | raises (CellMLException) |
Add a CellML element to this element.
Checking is performed to ensure that it is a type of element that is allowed in this type of element, and if it is not, an exception is raised. Checking is also performed to ensure that the element belongs in this Model, and that it is not already inserted into the document.
- Parameters
-
x The CellMLElement to add. CellMLException if the element cannot be added to this Element.
void cellml_api::CellMLElement::appendExtensionElement | ( | in ExtensionElement | x | ) |
Equivalent to insertExtensionElementAfter(last extension element, x).
- Parameters
-
x The element to append.
void cellml_api::CellMLElement::clearExtensionElements | ( | ) |
Remove all extension elements from this element.
CellMLElement cellml_api::CellMLElement::clone | ( | in boolean | deep | ) |
Clones a CellMLElement, and optionally all children.
This will not clone the contents of imported models.
- Parameters
-
deep If false, only clones the element. It will then have no children. If true, clones the element, and its children, which are added into the new element.
wstring cellml_api::CellMLElement::getExtensionAttributeNS | ( | in wstring | ns, |
in wstring | localName | ||
) |
Fetches an extension attribute.
- Parameters
-
ns The namespace of the extension attribute to fetch. localName The local name of the attribute to fetch.
UserData cellml_api::CellMLElement::getUserData | ( | in wstring | key | ) | raises (CellMLException) |
Retrieves user-supplied annotations previously set on this element.
- Parameters
-
key A string to identify the annotation.
- Returns
- The user-data associated with the key. CellMLException if no UserData is set for the given key.
void cellml_api::CellMLElement::insertExtensionElementAfter | ( | in ExtensionElement | marker, |
in ExtensionElement | newEl | ||
) |
Insert an element into the collection of extension elements without disturbing the order of the existing elements.
- Parameters
-
marker The element after which to insert the element. If this is nil, then the insertion will be at the start of the list. newEl The new element to insert.
void cellml_api::CellMLElement::prependExtensionElement | ( | in ExtensionElement | x | ) |
Equivalent to insertExtensionElementAfter(nil, x)
- Parameters
-
x The element to prepend.
void cellml_api::CellMLElement::removeByName | ( | in CellMLAttributeString | type, |
in CellMLAttributeString | name | ||
) |
Remove a CellML element by name, or do nothing if that element is not found.
- Parameters
-
type The type of element("variable", "component", etc...) name The name to remove.
void cellml_api::CellMLElement::removeElement | ( | in CellMLElement | x | ) |
Removes a CellML element from this element.
- Parameters
-
x The CellMLElement to remove.
void cellml_api::CellMLElement::removeExtensionAttributeNS | ( | in wstring | ns, |
in wstring | localName | ||
) |
Removes an extension attribute.
No action is taken if the attribute is not already present.
- Parameters
-
ns The namespace of the extension attribute to remove. localName The local name of the attribute to remove.
void cellml_api::CellMLElement::removeExtensionElement | ( | in ExtensionElement | x | ) |
Remove an extension element.
If the element is not found, do nothing.
- Parameters
-
x The element to remove.
void cellml_api::CellMLElement::replaceElement | ( | in CellMLElement | x, |
in CellMLElement | y | ||
) | raises (CellMLException) |
Removes a CellML element from this element, and replaces it with another CellML element.
CellMLException if the new element is of a type not allowed in this Element type, or belongs to the wrong Model.
- Parameters
-
x The CellMLElement to remove. y The CellMLElement to add.
void cellml_api::CellMLElement::replaceExtensionElement | ( | in ExtensionElement | x, |
in ExtensionElement | y | ||
) |
Find an extension element, and if it is found, replace it with another element.
- Parameters
-
x The element to find. y The element to replace x with.
void cellml_api::CellMLElement::setExtensionAttributeNS | ( | in wstring | ns, |
in wstring | qualifiedName, | ||
in wstring | value | ||
) |
Sets an extension attribute (adding it if it doesn't already exist, otherwise replacing it).
- Parameters
-
ns The namespace of the extension attribute to set. qualifiedName The qualified name of the attribute to set. value The value to set the attribute to.
void cellml_api::CellMLElement::setUserData | ( | in wstring | key, |
in UserData | data | ||
) |
Sets user-supplied annotations on this element.
These annotations are never read from an XML file or written out, but are for the application to use for any purpose it likes. Implementations in languages in which the ability to do this on any object is built in may choose not to implement this, provided that the implementation does not offer the possibility of remote access to the object. It is recommended that the key be a URL under the control of the person defining the meaning of the key. If the key already exists, then the existing user-data should be removed and the new user-data added.
- Parameters
-
key A string to identify the annotation. data The data to set, or nil to clear the UserData for a key.
Member Data Documentation
readonly attribute CellMLAttributeString cellml_api::CellMLElement::cellmlVersion |
The CellML version that this element corresponds to.
Can be the string "1.0" or "1.1". Other values are reserved for future use.
Definition at line 171 of file CellML_APISPEC.idl.
readonly attribute CellMLElementSet cellml_api::CellMLElement::childElements |
Get a list of all the child CellMLElements in this element.
Definition at line 229 of file CellML_APISPEC.idl.
attribute CellMLAttributeString cellml_api::CellMLElement::cmetaId |
This element's cmeta id (which may be defined on any CellML element).
Definition at line 176 of file CellML_APISPEC.idl.
readonly attribute ExtensionAttributeSet cellml_api::CellMLElement::extensionAttributes |
Fetches the set of all extension attributes, which can be used to iterate through the extension attributes.
Definition at line 340 of file CellML_APISPEC.idl.
readonly attribute ExtensionElementList cellml_api::CellMLElement::extensionElements |
The collection of extension elements associated with this CellML element.
The list is "live", and so it will automatically update as changes to the underlying DOM representation are made.
Definition at line 183 of file CellML_APISPEC.idl.
readonly attribute Model cellml_api::CellMLElement::modelElement |
The underlying model element.
Definition at line 276 of file CellML_APISPEC.idl.
readonly attribute CellMLElement cellml_api::CellMLElement::parentElement |
The parent element of this element...
Definition at line 271 of file CellML_APISPEC.idl.